db.orders.insert(
"id" : 1,
"item" : "MON1003",
"price" : 350,
"quantity" : 2,
"specs" :[ "27 inch", "Retina display", "1920x1080" ],
"type" : "Monitor"
specs
对应的value是数组格式
。
向集合inventory
新插入的数据 如下:
db.inventory.insert({ "id" : 1, "sku" : "MON1003", "type" : "Monitor", "instock" : 120,"size" : "27 inch", "resolution" : "1920x1080" })
db.inventory.insert({ "id" : 2, "sku" : "MON1012", "type" : "Monitor", "instock" : 85,"size" : "23 inch", "resolution" : "1280x800" })
db.inventory.insert({ "id" : 3, "sku" : "MON1031", "type" : "Monitor", "instock" : 60,"size" : "23 inch", "display_type" : "LED" })
查询的语句如下:
db.getCollection('orders').aggregate([
$unwind: "$specs"
db.getCollection('orders').aggregate([
$unwind: "$specs"
$lookup:
from: "inventory",
localField: "specs",
foreignField: "size",
as: "inventory_docs"
查询显示结果如下:
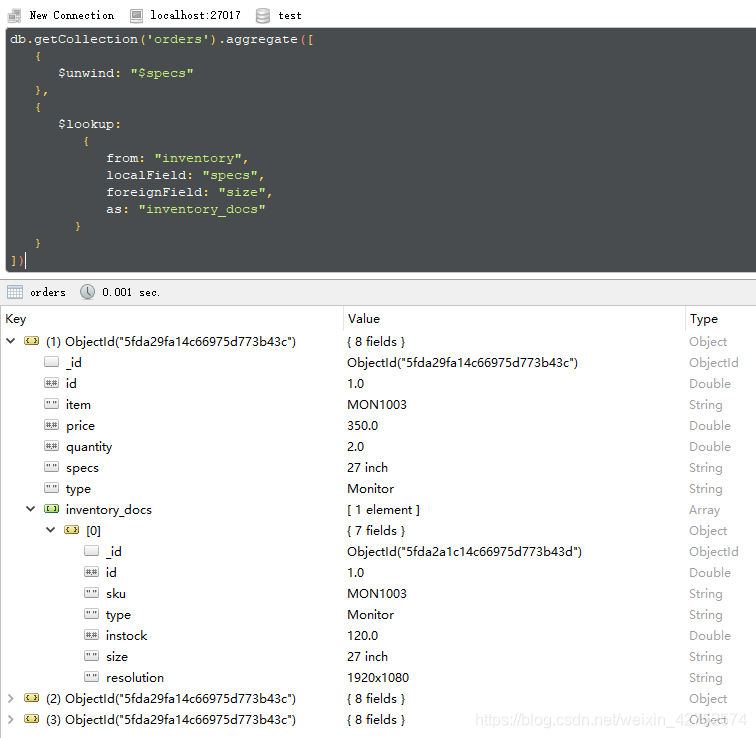
输出文档中的specs 对应的数据变成了字符串类型(原集合为数组)。 原因是 $unwind
$unwind: "$specs"
上面的结果前提下,只查询specs为"27 inch"的数据
db.getCollection('orders').aggregate([
$unwind: "$specs"
$lookup:
from: "inventory",
localField: "specs",
foreignField: "size",
as: "inventory_docs"
$match:{"specs": "27 inch"}
结果如下
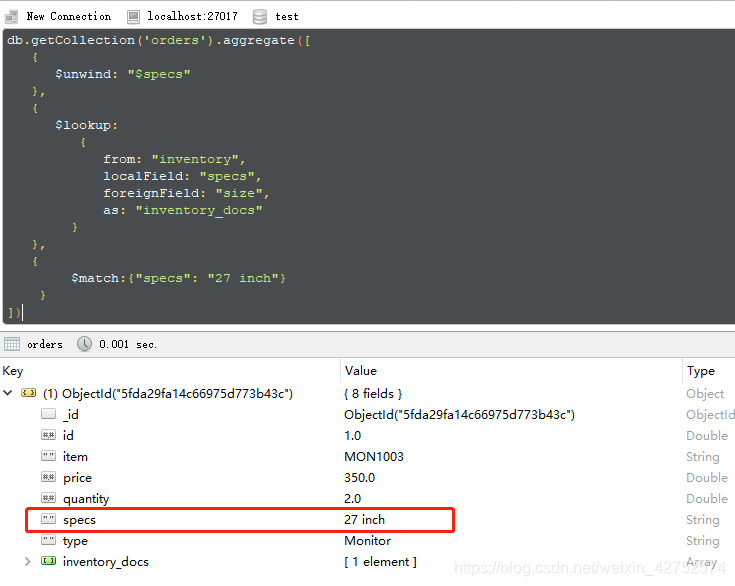
再上面的前提下,只输出item,inventory_docs字段
db.getCollection('orders').aggregate([
$unwind: "$specs"
$lookup:
from: "inventory",
localField: "specs",
foreignField: "size",
as: "inventory_docs"
$match:{"specs": "27 inch"}
$project: {item:1, inventory_docs:1}
最后一道题,在SQL中两表关联,每个表都有条件,那么在MongoDB中应该如何书写呢?
db.Rel_QQDetails.aggregate([
{ $match: {
ReconciliationId:CSUUID("bb54bee7-187f-4d38-85d7-88926000ac7a")
{ $lookup:
from: "Fct_QQStatements",
localField: "OrderId",
foreignField: "OrderStatementsId",
as: "inventory_docs"
{ $match : {
"inventory_docs.StatementsPriceException" :false
附加题,mongodb 集合间关联后更新,在MongoDB中应该如何书写呢?----借助 forEach 功能
由于篇幅限制(偷懒)
集合中的数据格式不再说明。
需求:集合QQ_OrderReturn 和 RelQQ_ReconciliationDetails 关联刷选,刷选符合条件,在更新QQ_OrderReturn的数据。
db.QQ_OrderReturn.aggregate([
{$match:{"Status" : 21}},
{$match:{"Disabled" : 0}},
{$match:{"JoinResponParty" : "合作方"}},
{$match:{ SupplierSellerName:"(合作营)ABC阳澄湖蟹"}},
$lookup:
from: "RelQQ_ReconciliationDetails",
localField: "OrderReturnId",
foreignField: "OrderId",
as: "inventory_docs"
{ $match : {"inventory_docs" : [ ]} }
]).forEach(function(item){
db.QQ_OrderReturn.update({"id":item.id},{$set:{"Status":NumberInt(0)}})
$unwind:将文档中的某一个数组类型字段拆分成多条,每条包含数组中的一个值。
db.orders.insert(
"id" : 1,
"item" : "MON1003",
"price" : 350,
"quantity" : 2,
"specs" :[ "27 inch", "Retina display", "1920x1080" ],
"type" : "Monitor"
对specs数组进行拆分:
db.orders.aggregate([
{$unwind:"$specs"}
拆分结果:
"id" : 1,
"item" : "MON1003",
"price" : 350,
"quantity" : 2,
"specs" : "27 inch",
"type" : "Monitor"
"id" : 1,
"item" : "MON1003",
"price" : 350,
"quantity" : 2,
"specs" :"Retina display",
"type" : "Monitor"
"id" : 1,
"item" : "MON1003",
"price" : 350,
"quantity" : 2,
"specs" :"1920x1080",
"type" : "Monitor"
使用$unwind
可以将specs数组
中的每个数据都被分解成一个文档,并且除了specs
的值不同外,其他的值都是相同的.
问题一:
在表A中有一个字段,类型为数组,里面存放的表B的ID字段。所以一条表A的数据对应复数条表B的数据。现在我每次只查询单条表A的数据,想通过$unwind和$lookup
关联查询,但返回的结果是一个数组,数组里面除了插入的表B的数据不同之外其他的数据都是重复的。
我希望达成的效果
是最终只返回一条表A的数据,这条数据中的那个数组里是我查询并插入的所有表B的数据,这样结果就不会有太多的重复,但我不知道该如何实现这样的效果?
问题二

第二个问题是,使用$lookup
后,会将子表的所有数据全都插入到主表中,但我只希望获取子表中特定的某几项数据,其余全都不查询,或是不显示。但$project
好像只对主表有用,不能删选掉插入的子表中的字段,想请教下应该如何才能在聚合管道里删选掉子表里的特定字段?
解决思路
因为Mongo操作我也不熟悉,就我目前对Mongo操作的了解得到的 解决思路如下,熟悉聚合管道相关的操作后,只要能从表里拿到数据,不管数据是否有多余的,想怎么改造,交给js实现,比较灵活
下面是问题涉及到的聚合管道操作实例,可以看看
希望可以帮到你
db.a.insert(
a1: 'hello',
a2: 'world',
a3: '!',
a4: ['001','002', '003']
db.b.insert(
id: '001',
b1: 'do',
b2: 'better',
b3: '!',
id: '002',
b1: 'do',
b2: 'better',
b3: '!',
id: '003',
b1: 'do',
b2: 'better',
b3: '!',
执行下面命令
db.getCollection('a').aggregate([
{$unwind: "$a4"}
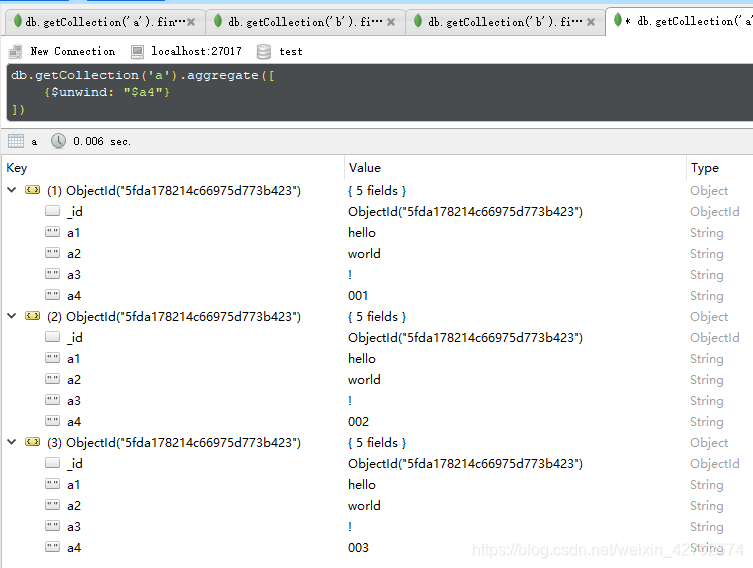
执行下面查询得到
db.getCollection('a').aggregate([
{$unwind: "$a4"},
{$match : {"a4" : "002"}}
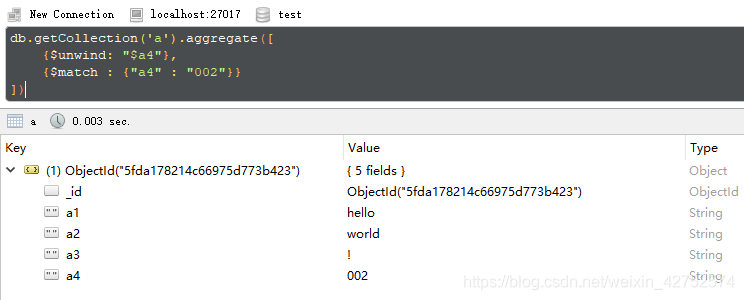
执行下面的查询得到
db.getCollection('a').aggregate([
{$unwind: "$a4"},
{$match : {"a4" : "002"}},
{$project: {a2:1}}
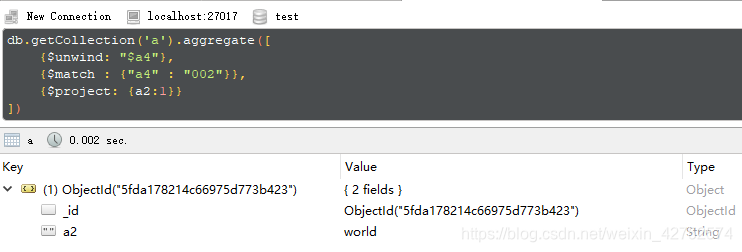
执行下面的查询得到
db.getCollection('a').aggregate([
{$unwind: "$a4"},
$lookup:
from: "b",
localField: "a4",
foreignField: "id",
as: "new"
谢谢你阅读到了最后~
期待你关注、收藏、评论、点赞~
让我们一起 变得更强
mongodb、mongoose相关
MongoDB 常用命令汇总 该有的都有
详解MongoDB中的多表关联查询($lookup)管道的概念聚合框架$lookup的功能及语法主要功能基本语法例子说明$unwind的功能及语法例子你越是认真生活,你的生活就会越美好——弗兰克·劳埃德·莱特《人生果实》经典语录mongodb中文文档mongoose框架文档管道的概念管道在Unix和Linux中一般用于将当前命令的输出结果作为下一个命令的参数。MongoDB的聚合管道将MongoDB文档在一个管道处理完毕后将结果传递给下一个管道处理。管道操作是可以重复的。聚合框架Mon
MongoDBMongoDB 聚合管道聚合管道概述需要注意的几个问题:聚合管道操作符$project 指定文档显示的方式连接字段$concat用于连接字符串(参数必须是字符串类型数组)对字段进行分解 $substr$match相当于find第一个参数,用于过滤文档$limit $skip $sort的使用$unwind
MongoDB 聚合管道
聚合管道概述
聚合管道由阶段组成
每个阶段由阶段操作符来对文档进行相应的处理
待处理的文档会流经各个阶段,最终完成计算
db.集合名.aggregate([{}])
mongo aggregate操作使用$lookup,$unwind,$project,$group操作符,执行多表连接查询,提取多表指定字段,对指定字段进行分组求和得到结果:
db.getCollection('DeviceDetailInfo_20191230').aggregate([
{$lookup:{from:'DeviceDetailInfo_20191229',localField...
from: <collection to join>,
localField: <field from the input documents>,
foreignField: <field from the documents of the "from" collection>,
as: <output array field>
NodeJs操作MongoDB之多表查询($lookup)与常见问题
一,方法介绍
aggregate()方法来对数据进行聚合操作。aggregate()方法的语法如下
1 aggregate(operators,[options],callback)
operators参数是如表1所示的聚合运算符的数组,它允许你定义对数据执行什么汇总操作。options参数允许你设置r...
1. 基本操作
$group可以用来对文档进行分组,比如我想将订单按照城市进行分组,并统计出每个城市的订单数量:
db.sang_collect.aggregate({$group:{_id:"$orderAddressL",count:{$sum:1}}})
我们将要分组的字段传递给$group函数的_id字段,然后每当查到一个,就给count加1,这样就可以统计出每个城市的订单数量。
2. 算术操作符
通过算术操作符我们可以对分组后的文档进行求和或者求平均数。比如我想计算每个城市订
MongoDB中的聚合(aggregate)查询($lookup、$unwind、$match、$project、$skip、$limit以及$sort操作符的使用)
文章目录一。概念二。集合示例准备三。各个操作符的用法1.$lookup2.$match3.$unwind4.$project5.$limit6.$skip7.$group8.$sort
管道的概念
管道在Unix和Linux中一般用于将当前命令的输出结果作为下一个命令的参数。
MongoDB的聚合管道将MongoDB文档在一个管道处理完毕后将结果传递给下一个管道处理。管道操作是可以重复的。
聚合框架
MongoDB中聚合(aggregate)主要用于处理数据(诸如统计平均值,求和等),并返回计算
用于过滤数据,只输出符合条件的文档使用MongoDB的标准查询操作例1:查询年龄大于20的学生db.stu.aggregate([
{$match:{age:{$gt:20}}}
例2:查询年龄大于20的男生、女生人数
db.stu.aggregate([
{$match:{age:{$gt:20}}},
{$group:{_id:'$ge
假设我们有两个集合:orders(订单)和 customers(客户),它们之间通过一个共同的字段 customerId(客户ID)进行关联。我们需要在 orders 集合中查询所有的订单,并将与之对应的客户信息也一并查询出来,最终的结果是将客户信息作为嵌套文档添加到订单文档中。
下面是使用 MongoDB 聚合框架进行多表聚合查询的代码:
db.orders.aggregate([
//使用lookup进行关联查询
$lookup: {
from: "customers",//关联的表名
localField: "customerId",//本地关联字段
foreignField: "_id",//外部关联字段
as: "customer"//查询结果别名
//使用unwind将customer数组展开
$unwind: "$customer"
//使用match进行过滤
$match: {
"customer.name": "张三"
//使用project进行字段投影
$project: {
_id: 1,
orderNo: 1,
customer: {
name: 1,
mobile: 1
在以上代码中,我们使用了 $lookup 操作符进行多表关联查询,并指定了 from、localField、foreignField 和 as 参数。接着使用 $unwind 操作符将查询结果中的 customer 数组展开成多个文档。然后使用 $match 操作符进行过滤,最后使用 $project 操作符进行字段投影,只保留需要的字段。