如果我们想改变QMap的key的排序规则,则需要提供operator<()
QMap’s key type must provide operator<(). QMap uses it to keep its items sorted, and assumes that two keys x and y are equal if neither x < y nor y < x is true.
Qt帮助文档给的例子:
#ifndef EMPLOYEE_H
#define EMPLOYEE_H
class Employee
public:
Employee() {}
Employee(const QString &name, const QDate &dateOfBirth);
...
private:
QString myName;
QDate myDateOfBirth;
inline bool operator<(const Employee &e1, const Employee &e2)
if (e1.name() != e2.name())
return e1.name() < e2.name();
return e1.dateOfBirth() < e2.dateOfBirth();
#endif
也可以把operator<写成类成员函数。
自己写的一个例子:
在Qt中新建一个控制台程序:
main.cpp中
#include <QCoreApplication>
#include <QMap>
#include <QDebug>
class MyString
public:
explicit MyString(const QString &str_) : str(str_) {}
QString str;
bool operator < (const MyString& other) const
return str > other.str;
int main(int argc, char *argv[])
QCoreApplication a(argc, argv);
QMap<MyString,int> stringMap;
stringMap.insert(MyString("aa"), 4);
stringMap.insert(MyString("bb"), 3);
stringMap.insert(MyString("cc"), 2);
stringMap.insert(MyString("dd"), 1);
QMapIterator<MyString, int> iter(stringMap);
while (iter.hasNext()) {
iter.next();
qDebug() << iter.key().str << ": " << iter.value() << Qt::endl;
return a.exec();
运行结果:
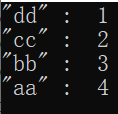
就变成了key是按照QString进行降序排列。
如果代码改成这样:
class MyString
public:
explicit MyString(const QString &str_) : str(str_) {}
QString str;
bool operator < (const MyString& other) const
return str < other.str;
我再写一个按照int降序排序的例子:
#include <QCoreApplication>
#include <QMap>
#include <QDebug>
class MyInt
public:
explicit MyInt(const int &value_) : value(value_) {}
int value;
bool operator < (const MyInt& other) const
return value > other.value;
int main(int argc, char *argv[])
QCoreApplication a(argc, argv);
QMap<MyInt, QString> intMap;
intMap.insert(MyInt(1), QString("aa"));
intMap.insert(MyInt(2), QString("bb"));
intMap.insert(MyInt(3), QString("cc"));
intMap.insert(MyInt(4), QString("dd"));
QMapIterator<MyInt, QString> iter(intMap);
while (iter.hasNext()) {
iter.next();
qDebug() << iter.key().value << ": " << iter.value();
return a.exec();
运行结果:
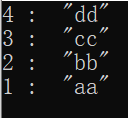
以上只是为了让举例的代码简单明了,没有写成get和set的方式对类成员变量进行操作,在项目中建议写出get和set的方法对类成员变量进行操作。
QMap的默认排序是按照key的升序进行排序。如果我们想改变QMap的key的排序规则,则需要提供operator<()QMap’s key type must provide operator<(). QMap uses it to keep its items sorted, and assumes that two keys x and y are equal if neither x < y nor y < x is true.Qt帮助文档给的例子: #ifnd
Map排序 根据key排序
map的key是数字,我在应用的时候是根据固定的规则吧key设置的是顺序_类型作为map的key值,在判断的时候截取进行的比较
public int compare(String s1, String s2) {
Integer int1 = Integer.valueOf(s1.split("_")[0]);
Integer int2 = Integer.valueOf(s2.split("_")[0]);
QVector<QPointF> ReadSonicPoint::sortSonicPoint(QVector<QPointF> befPointVector) //把x轴的值从小到大排序
QVector<QPointF> modPointVector;
QMap<double, double>sonicMap; //利用QMap自动排序
QMap原型为class QMap <K,T>,其中K表示键,T表示值,K和T属于映射关系.
QMap会根据K来自动进行升序键排序
QMap中的K类型必须重载operator <
QMap常用函数如下:
const Key QMap::key ( const T & value );
//通过值来找键,若未找到则返回0,由于只对K键进行排序,所以该函数不是快速查找
const T QMap::value ( c
Map<LocalDateTime, List<ExerciseReport>> twelveReportMap = twelveReportDataList.stream()
.collect(Collectors.groupingBy(ExerciseReport::getCreateDate));
System.out.println(
有一个QMap<QString,qreal> map,存储的数据为名字对应值,如: apple -> 12.2 , orange-> 23.3,banana->10.5。 现在需要按照map元素值的升序的顺序获取QMap中的所有元素。
也就是希望获得的结果是:banana,10.5 -> apple,12.2 -> orange,23.3 。
qSort可..
要在 QMap 中插入自定义数据结构,首先需要确保该数据结构实现了比较运算符,以便在 QMap 中进行排序和查找。比较运算符可以通过重载操作符实现,例如:
struct MyStruct {
int id;
QString name;
bool operator<(const MyStruct& other) const {
return id < other.id;
以上代码定义了一个包含 id 和 name 两个成员变量的自定义数据结构 MyStruct,并重载了小于运算符,以便在 QMap 中进行排序和查找。
接下来,可以通过 QMap 的 insert 或 insertMulti 方法插入自定义数据结构。例如:
QMap<int, MyStruct> myMap;
MyStruct myStruct1 = { 1, "Alice" };
MyStruct myStruct2 = { 2, "Bob" };
myMap.insert(1, myStruct1);
myMap.insert(2, myStruct2);
以上代码创建了一个 QMap 对象 myMap,并向其中插入了两个 MyStruct 对象。其中,键为整数类型,值为 MyStruct 类型。
需要注意的是,如果自定义数据结构中的比较运算符发生了变化,可能会导致 QMap 中的排序和查找结果不一致。因此,在修改自定义数据结构时需要谨慎考虑。