How to Use publicExponent as 65537 in RSA-OAEP Algorithm in JavaScript
publicExponent
as 65537 in the RSA-OAEP algorithm in JavaScript.
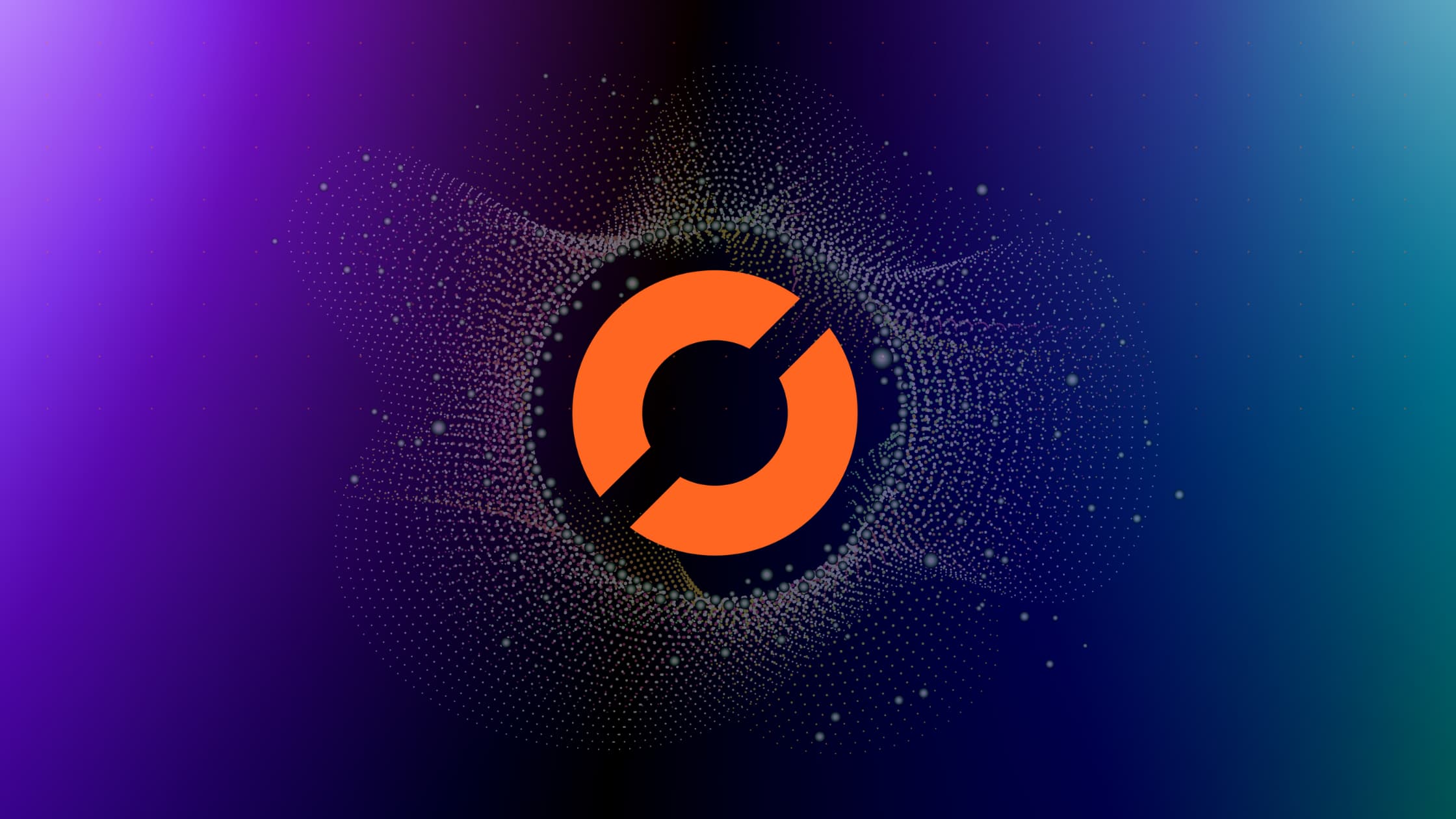
How to Use publicExponent as 65537 in RSA-OAEP Algorithm in JavaScript
Introduction
As a data scientist or software engineer working with encryption algorithms, you may come across the RSA-OAEP
algorithm
. RSA-OAEP (Optimal Asymmetric Encryption Padding) is a widely used encryption scheme that provides secure communication and data transfer. In this article, we will explore how to use the
publicExponent
as 65537 in the RSA-OAEP algorithm in JavaScript.
Understanding RSA-OAEP Algorithm
Before diving into the specifics of using
publicExponent
as 65537 in the RSA-OAEP algorithm, let’s briefly understand the basics of RSA and OAEP.
RSA is a public-key encryption algorithm widely used for secure data transmission. It utilizes a pair of keys: a public key for encryption and a private key for decryption. The RSA algorithm is based on the mathematical problem of factoring large composite numbers.
OAEP, on the other hand, stands for Optimal Asymmetric Encryption Padding. It is a padding scheme that enhances the security of RSA encryption by adding randomization and hashing functions. OAEP helps protect against chosen-ciphertext attacks and other vulnerabilities.
Using publicExponent as 65537 in JavaScript
To use
publicExponent
as 65537 in the RSA-OAEP algorithm in JavaScript, you can leverage cryptographic libraries such as
crypto
or
node-rsa
. These libraries provide convenient methods to generate RSA key pairs and perform encryption and decryption operations.
Here is a step-by-step guide on how to use
publicExponent
as 65537 in the RSA-OAEP algorithm using the
crypto
library in JavaScript:
Step 1: Install Required Libraries
First, you need to install the
crypto
library. You can do this by running the following command:
npm install crypto
Step 2: Generate RSA Key Pair
Next, you need to generate an RSA key pair. The key pair consists of a public key and a private key. In this example, we will focus on the public key and set the
publicExponent
to 65537. Here’s how you can generate the key pair:
const crypto = require('crypto');
const { publicKey, privateKey } = crypto.generateKeyPairSync('rsa', {
modulusLength: 2048,
publicExponent: 65537,
Step 3: Encrypting Data
Once you have the RSA public key, you can use it to encrypt your data. The
crypto
library provides a method called
publicEncrypt
that performs the encryption operation using the RSA-OAEP algorithm. Here’s an example of encrypting data using the RSA public key:
const data = 'Hello, World!';
const encryptedData = crypto.publicEncrypt(
key: publicKey,
padding: crypto.constants.RSA_PKCS1_OAEP_PADDING,
oaepHash: 'sha256',
Buffer.from(data, 'utf8')
Step 4: Decrypting Data
To decrypt the encrypted data, you will need the corresponding RSA private key. The
crypto
library provides a method called
privateDecrypt
for decryption. Here’s an example of decrypting the data:
const decryptedData = crypto.privateDecrypt(
key: privateKey,
padding: crypto.constants.RSA_PKCS1_OAEP_PADDING,
oaepHash: 'sha256',