![]() |
奔放的蜡烛 · 深圳市开放职业技术学校(龙岗区)_考点地址_ ...· 5 月前 · |
![]() |
稳重的猴子 · 关于electron的开发应用路径和生产路径 ...· 6 月前 · |
![]() |
八块腹肌的柑橘 · 爱奇艺尖叫之夜2023嘉宾有哪些(完整名单) ...· 8 月前 · |
![]() |
发怒的芒果 · 危险病患by幼稚鬼_危险病患by幼稚鬼写的小 ...· 1 年前 · |
Spring WebFlux is a part of the Spring Framework that provides reactive programming support for web applications. It introduces reactive types like Mono and Flux publishers which are fundamental to its programming model. Mono and Flux play crucial roles in reactive programming. The reactive programming introduces a lot of operators to handle the business logic like flatmap, onErrorResume, map, zip, just, and other reactor operators. In this article, we explain what flatmap and switchIfEmpty.
To understand this content, you should know the topics below are easy to understand.
The flatmap is a operator is an operator in reactive programming particularly in Project Reactor and RxJava, that transforms each element emitted by a source publisher into new publisher then flattens these inner publisher instance into a single publisher and It allows asynchronous processing of each element and merges the result.
We use flatmap operator on publisher to handle the business means It can handle every event in the publisher. Below we provide the different situations to use flatmap operator in reactive programming.
The switchIfEmpty is a another operator in the reactive programming and It is used to provide an alternative sequence if the source like publisher completes without emitting any Items. It essentially “switches” to a fallback Publisher when the original Publisher is empty.
Here, we provide examples for flatmap and switchIfEmpty operators with Mono and Flux publishers. Basically the switchIfEmpty is used when publisher emits nothing.
In this example, we write a simple program to explain the flatmap and switchIfEmpty with Mono publisher. Below we provide the example for your reference.
package com.app;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import reactor.core.publisher.Mono;
@SpringBootApplication
public class PublishersApplication {
public static void main(String[] args) {
SpringApplication.run(PublishersApplication.class, args);
Mono<String> msg = Mono.just("Hello Geeks");
msg.flatMap(msgs -> {
return Mono.just("Hello, " + msgs + "!");
}).switchIfEmpty(Mono.just("No data available")).subscribe(System.out::println);
- In the above program, first we create Mono publisher with String type and by using Mono.just().
- We provide the data to the publisher after this we use flatmap on Mono object, and this flatmap return a publisher.
- Immediately on flatmap we use switchIfEmpty operator. If publisher is empty the switchIfEmpty returns the No data available message other wise It returns Hello, Hello Geeks!
Output:
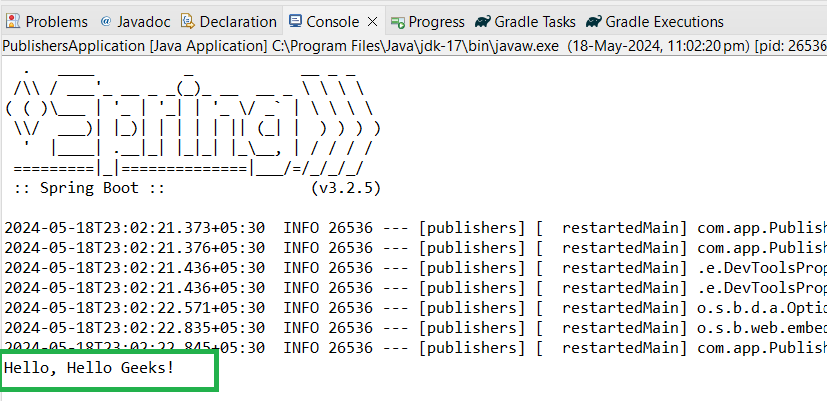
flatmap and switchIfEmpty with Flux publisher
In this example, we write a simple program to explain the flatmap and switchIfEmpty with Flux publisher. Below we provide the example for your reference.
package com.app;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
@SpringBootApplication
public class PublishersApplication {
public static void main(String[] args) {
SpringApplication.run(PublishersApplication.class, args);
Flux<String> names = Flux.just("John", "Marry", "Micky", "Hani");
names.flatMap(name -> {
return Mono.just("Hello, " + name + "!");
}).switchIfEmpty(Mono.just("No names available")).subscribe(System.out::println);
- In the above program, first we create Flux publisher with String type and by using Flux.just().
- We provide the data to the publisher after this we use flatmap on Flux object, and this flatmap return a publisher.
- Immediately on flatmap we use switchIfEmpty operator. If publisher is empty the switchIfEmpty returns the No names available message and then prints each names to the console.
Output:
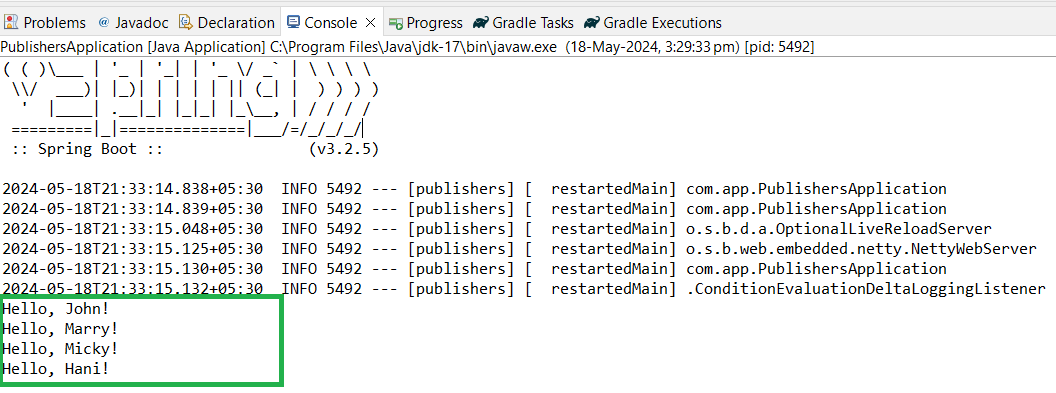
Spring Boot - Reactive Programming Using Spring Webflux Framework
In this article, we will explore React programming in Spring Boot, Reactive programming is an asynchronous, non-blocking programming paradigm for developing highly responsive applications that react to external stimuli. What is Reactive ProgrammingIn reactive programming, the flow of data is asynchronous through push-based publishers and subscriber
Spring Cloud Stream - Functional and Reactive
Spring Cloud Stream is a Spring framework that simplifies creating event-driven microservices. It uses functional programming constructs for message processing logic, often using annotated methods within a class and reactive programming tools like Reactor for asynchronous and reactive processing. Maven Dependencies<dependency> <groupId>
Testing Spring WebFlux Reactive CRUD Rest APIs Using WebTestClient
Spring Boot is one of the famous frameworks for Back-end development. The Spring Boot Community Developed the Spring Reactive Web Framework. This Reactive Framework is available from Spring Boot 5.0 and Above versions only. The Spring Reactive allows developers to build Asynchronous, Non-Blocking, and Event-Driven Web Applications. When compared wi
Spring WebFlux Reactive CRUD REST API Example
Spring WebFlux can be defined as the reactive programming framework provided by the Spring ecosystem for the building of asynchronous, non-blocking, and event-driven applications and it can be designed to handle a large number of concurrent connections while consuming less resources. Key Terminologies:Mono and Flux: Mono can represent the publisher
Router Functions in Spring Reactive
Spring Reactive programming is an asynchronous and non-blocking paradigm for developing highly responsive applications. It enables developers to build applications that handle high-load traffic without compromising performance. Spring WebFlux, a part of the Spring Framework, supports reactive programming with non-blocking, backpressure-enabled type
Building Reactive Microservices with Spring WebFlux
This article will teach us how to build reactive microservices with Spring WebFlux using a related example. Here, we create a student microservice to handle students and their data. For understanding purposes, we provide basic features and functionalities such as adding a student, searching for a student, deleting a student, and getting all student
Spring WebFlux Application Integration with Reactive Messaging System Kafka
Spring WebFlux is the framework for building reactive applications on the JVM. It is useful for reactive programming and makes it easier to build asynchronous, non-blocking and event-driven applications. Apache Kafka is a distributed streaming platform which allows us to publish and subscribe to streams of the records in a fault-tolerant way and pr
Reactive JWT Authentication Using Spring WebFlux
JSON Web Token (JWT) authentication is a popular method for securing APIs in microservices architectures. With Spring WebFlux, the reactive web framework, we can create highly scalable and responsive applications. In this article, we will guide you on how to implement JWT authentication in a reactive Spring WebFlux application. JWT is a compact and
Migrating a Spring Boot Application from Spring Security 5 to Spring Security 6
Spring Security is a powerful authentication and access control framework for Java applications specially for those built with the Spring Framework. With the release of Spring Security 6, several enhancements and changes have been introduced to simplify the security configuration and provide better performance and security features. This article ai
Monitoring and Logging for Reactive Applications
Reactive applications are designed to be responsive, resilient, elastic, and message-driven. Monitoring and logging are the crucial aspects of maintaining and troubleshooting these applications. They can provide insights into the application performance, help detect issues early, and ensure smooth operation. This article explores how to set up the
- Company
- About Us
- Legal
- In Media
- Contact Us
- Advertise with us
- GFG Corporate Solution
- Placement Training Program
- GeeksforGeeks Community
- DSA
- Data Structures
- Algorithms
- DSA for Beginners
- Basic DSA Problems
- DSA Roadmap
- Top 100 DSA Interview Problems
- DSA Roadmap by Sandeep Jain
- All Cheat Sheets
- Computer Science
- Operating Systems
- Computer Network
- Database Management System
- Software Engineering
- Digital Logic Design
- Engineering Maths
- Software Development
- Software Testing
- System Design
- High Level Design
- Low Level Design
- UML Diagrams
- Interview Guide
- Design Patterns
- OOAD
- System Design Bootcamp
- Interview Questions
We use cookies to ensure you have the best browsing experience on our website. By using our site, you
acknowledge that you have read and understood our
Cookie Policy &
Privacy Policy
Got It !
Please go through our recently updated Improvement Guidelines before submitting any improvements.
This improvement is locked by another user right now. You can suggest the changes for now and it will be under 'My Suggestions' Tab on Write.
You will be notified via email once the article is available for improvement.
Thank you for your valuable feedback!
Please go through our recently updated Improvement Guidelines before submitting any improvements.
Suggest Changes
Help us improve. Share your suggestions to enhance the article. Contribute your expertise and make a difference in the GeeksforGeeks portal.