单测:Google Test框架
原创介绍
Google Test是一个流行的C++单元测试框架,它提供了丰富的断言和测试工具,用于编写和运行单元测试。基于流行的 xUnit 架构
编译
1、源码
源码下载比较简单:
git clone https://github.com/google/googletest.git
源码分为四块
文件夹 |
说明 |
---|---|
ci |
这是Google Test为各个平台提供的快速部署脚本文件夹 |
docs |
这是google test框架的文档 |
googlemock |
这是Google Mock的源码文件夹,它是Google Test的一个扩展,用于编写和运行C++的模拟对象测试。Google Mock提供了模拟对象和行为的功能,用于进行单元测试。 |
googletest |
这是Google Test的核心代码所在的文件夹。它包含了Google Test框架的实现,包括测试框架的主要功能和断言宏等。 |
2、环境
工具:Visual Studio 2022 专业版
安装必要工具:工具 - 获取工具和功能
必要组件:
- 用于Windows的C++ CMake工具
- Google Test 测试适配器
3、配置
配置指定编译选项:
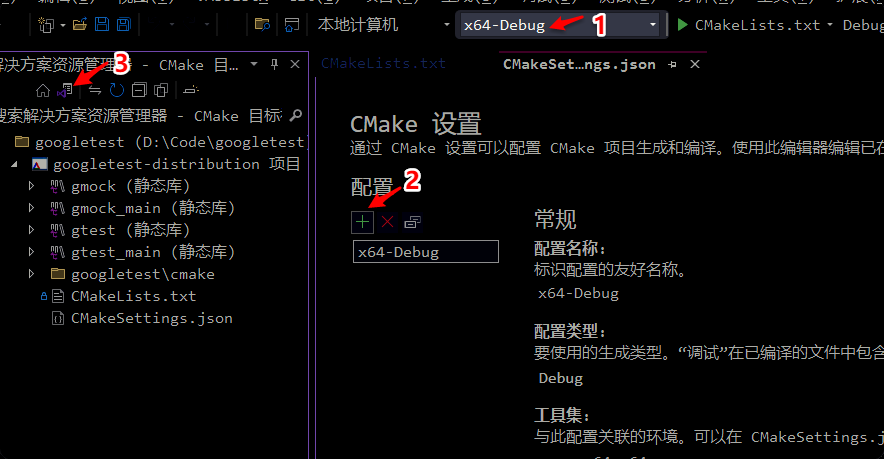
以x86-debug为例进行配置,新增后基本上保持默认配置即可,项目属性右键选择 安装
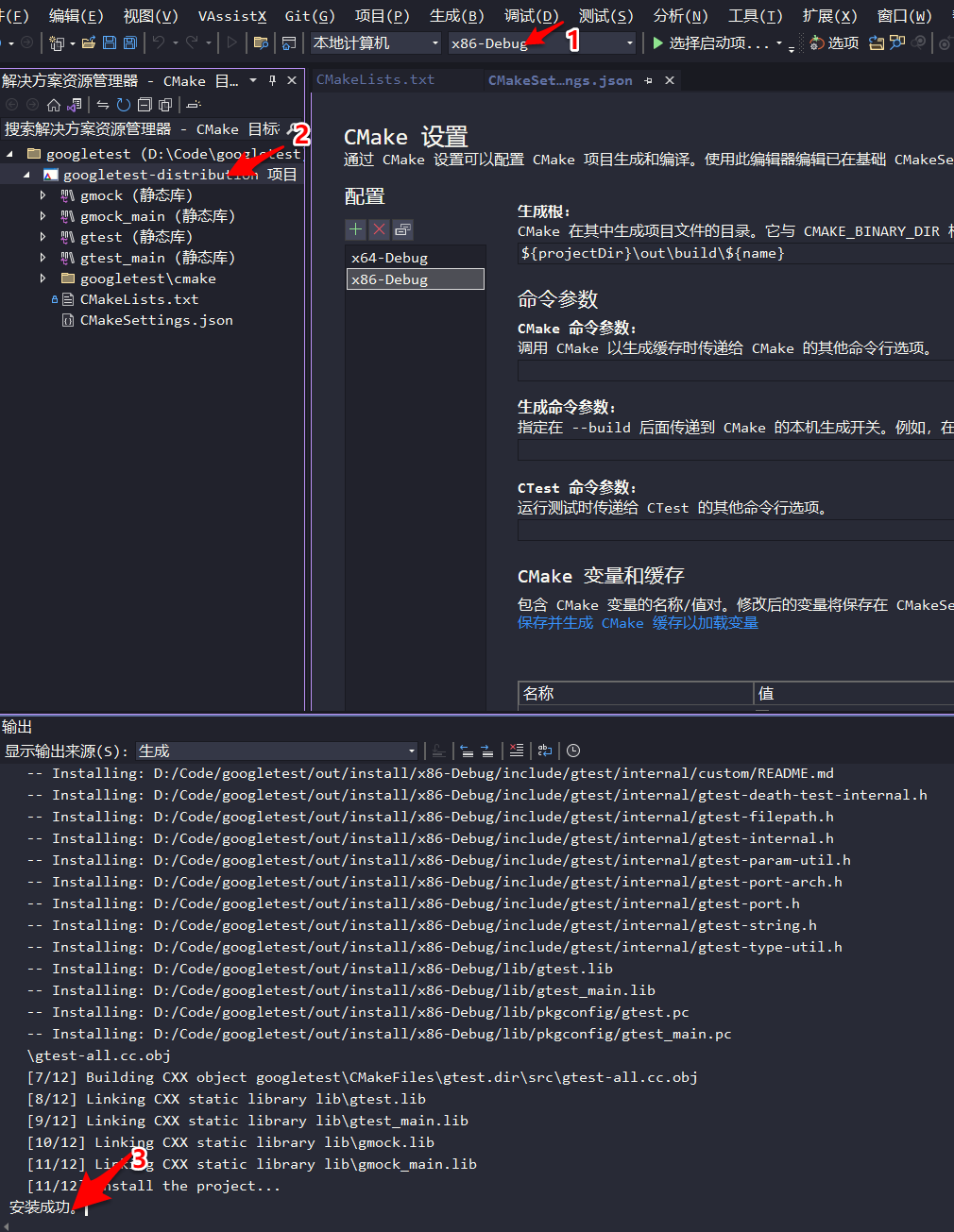
显示安装成功,即可在默认输出路径:
${projectDir}\out\build\${name}
默认安装路径:
${projectDir}\out\install\${name}
默认是不编译除了gtest和gmock之外的项目
4、编译
产物路径:
${projectDir}\out\install\x86-Debug\lib
头文件路径:
${projectDir}\out\install\x86-Debug\include
如果想编译其他项目,以gtest_build_samples为例,如下勾选,ctrl+S保存,即可发现左边方案选项卡新增sample的编译
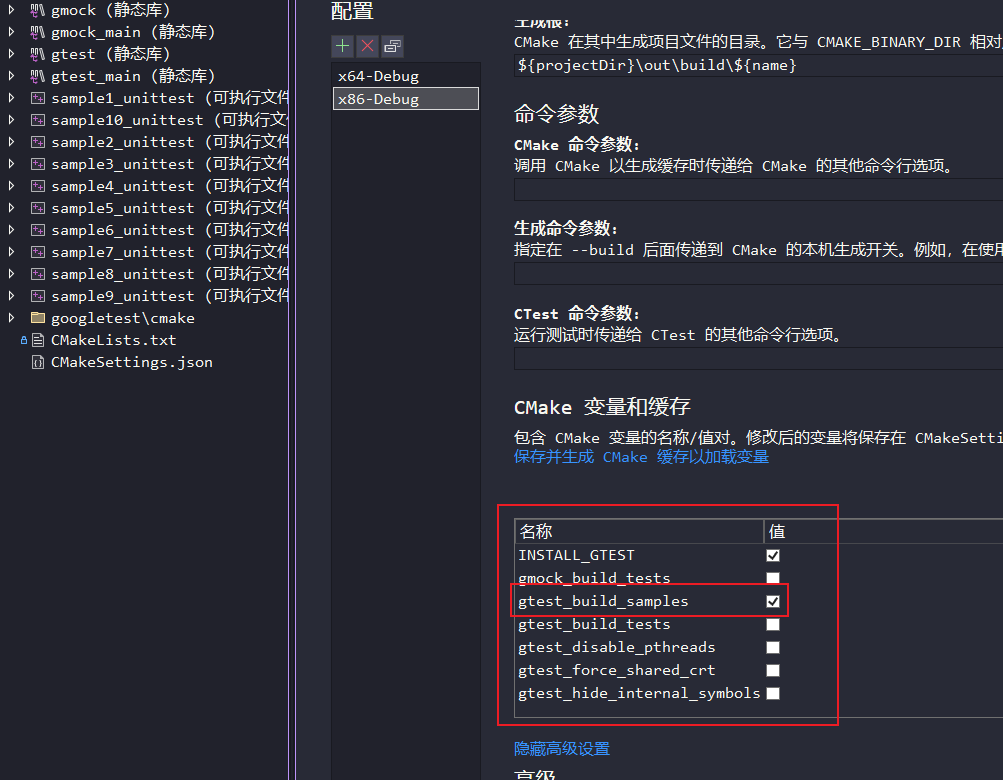
右键sample1进行生成
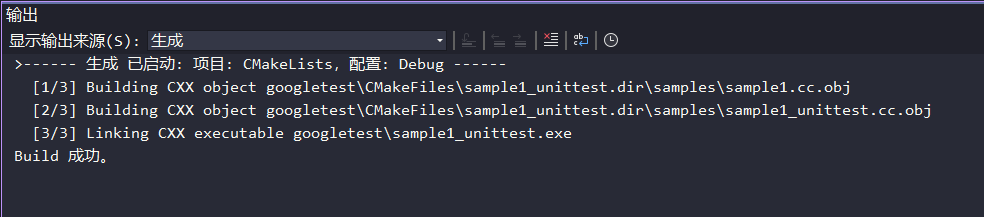
在
${projectDir}\out\build\x86-Debug\googletest
目录就可以找到生成可执行文件,命令行执行可以看到Test结果
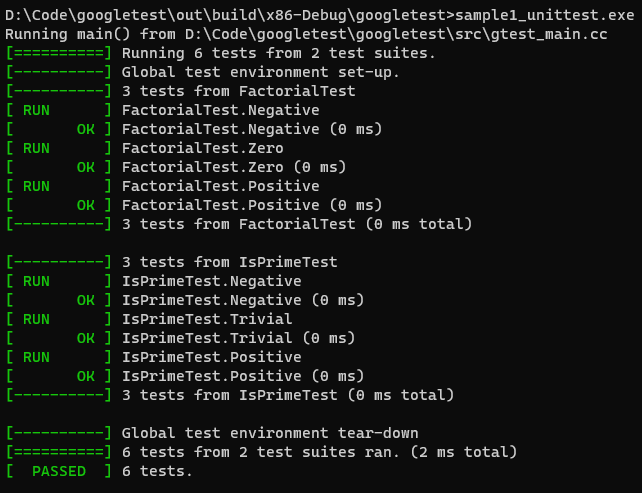
5、使用
参考官方给的sample可以很容易编写出一个基于Cmake的单元测试样例,可是如何集成到Visual Studio中使用此测试框架呢?
新建VS的全新控制台工程,迁移官方Sample1,修改编译配置(这里采用静态链接方式使用)
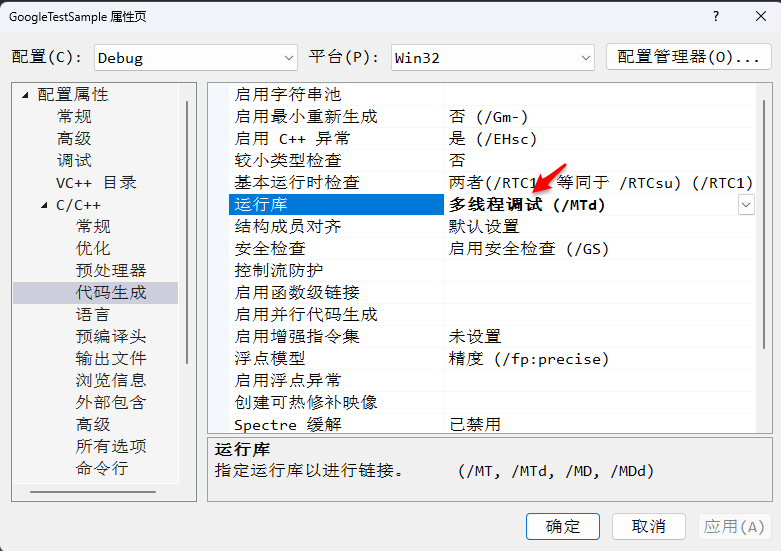
设置路径:
引入产物路径:
${projectDir}\out\install\x86-Debug\lib
引入头文件路径:
${projectDir}\out\install\x86-Debug\include
引入gtest.lib,main函数如下:
/************************************************************************/
/*@File Name : GoogleTestSample.cpp
/*@Created Date : 2023/12/29 0:20
/*@Author : Leal
/*@Description :
/************************************************************************/
#include <iostream>
#include "gtest/gtest.h"
#include "testSample.h"
using ::testing::InitGoogleTest;
using ::testing::Test;
// Tests factorial of negative numbers.
TEST(FactorialTest, Negative) {
// This test is named "Negative", and belongs to the "FactorialTest"
// test case.
EXPECT_EQ(1, Factorial(-5));
EXPECT_EQ(1, Factorial(-1));
EXPECT_GT(Factorial(-10), 0);
// Tests factorial of 0.
TEST(FactorialTest, Zero) { EXPECT_EQ(1, Factorial(0)); }
// Tests factorial of positive numbers.
TEST(FactorialTest, Positive) {
EXPECT_EQ(1, Factorial(1));
EXPECT_EQ(2, Factorial(2));
EXPECT_EQ(6, Factorial(3));
EXPECT_EQ(40320, Factorial(8));
// Tests IsPrime()
// Tests negative input.
TEST(IsPrimeTest, Negative) {
// This test belongs to the IsPrimeTest test case.
EXPECT_FALSE(IsPrime(-1));
EXPECT_FALSE(IsPrime(-2));
EXPECT_FALSE(IsPrime(INT_MIN));
// Tests some trivial cases.
TEST(IsPrimeTest, Trivial) {
EXPECT_FALSE(IsPrime(0));
EXPECT_FALSE(IsPrime(1));
EXPECT_TRUE(IsPrime(2));
EXPECT_TRUE(IsPrime(3));
// Tests positive input.
TEST(IsPrimeTest, Positive) {
EXPECT_FALSE(IsPrime(4));
EXPECT_TRUE(IsPrime(5));
EXPECT_FALSE(IsPrime(6));
EXPECT_TRUE(IsPrime(23));
int main(int argc, char** argv) {
InitGoogleTest(&argc, argv);
return RUN_ALL_TESTS();
}
注意拷贝待测试的函数头文件和cpp
/************************************************************************/
/*@File Name : testSample.cpp
/*@Created Date : 2023/12/29 0:22
/*@Author : Leal
/*@Description :
/************************************************************************/
// A sample program demonstrating using Google C++ testing framework.
#include "testSample.h"
// Returns n! (the factorial of n). For negative n, n! is defined to be 1.
int Factorial(int n) {
int result = 1;
for (int i = 1; i <= n; i++) {
result *= i;
return result;
// Returns true if and only if n is a prime number.
bool IsPrime(int n) {
// Trivial case 1: small numbers
if (n <= 1) return false;
// Trivial case 2: even numbers
if (n % 2 == 0) return n == 2;
// Now, we have that n is odd and n >= 3.
// Try to divide n by every odd number i, starting from 3
for (int i = 3;; i += 2) {
// We only have to try i up to the square root of n
if (i > n / i) break;
// Now, we have i <= n/i < n.
// If n is divisible by i, n is not prime.
if (n % i == 0) return false;
// n has no integer factor in the range (1, n), and thus is prime.
return true;
}
/************************************************************************/
/*@File Name : testSample.h
/*@Created Date : 2023/12/29 0:22
/*@Author : Leal
/*@Description :
/************************************************************************/
// A sample program demonstrating using Google C++ testing framework.
#ifndef GOOGLETEST_SAMPLES_SAMPLE1_H_
#define GOOGLETEST_SAMPLES_SAMPLE1_H_
// Returns n! (the factorial of n). For negative n, n! is defined to be 1.
int Factorial(int n);
// Returns true if and only if n is a prime number.
bool IsPrime(int n);
#endif // GOOGLETEST_SAMPLES_SAMPLE1_H_
编译通过,运行即可
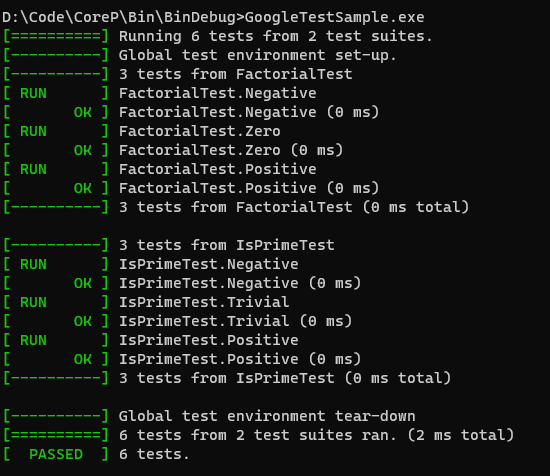
生成PDB
另:直接Cmake进行安装,是没有pdb生成,如若需要,则需要去源码下找到生成sln文件打开
D:\Code\googletest\build\googletest-distribution.sln
右键解决方案进行生成解决方案,有以下配置:(默认是Win32,需要64位需要调整Cmake属性重新生成sln文件)
配置说明,按需选用
配置 |
说明 |
---|---|
Debug |
默认自带PDB,关闭优化 |
Release |
默认不带PDB,开启/O2优化(优选速度) |
RelWithDebInfo |
默认带PDB,开启/O2优化(优选速度) |
MinSizeRel |
默认不带PDB,开启/O1优化(优选大小) |
6、Visual Studio创建Google Test项目
利用Visual studio中Google Test 测试适配器这个组件提供的能力,可以直接在visual studio中创建Google Test项目
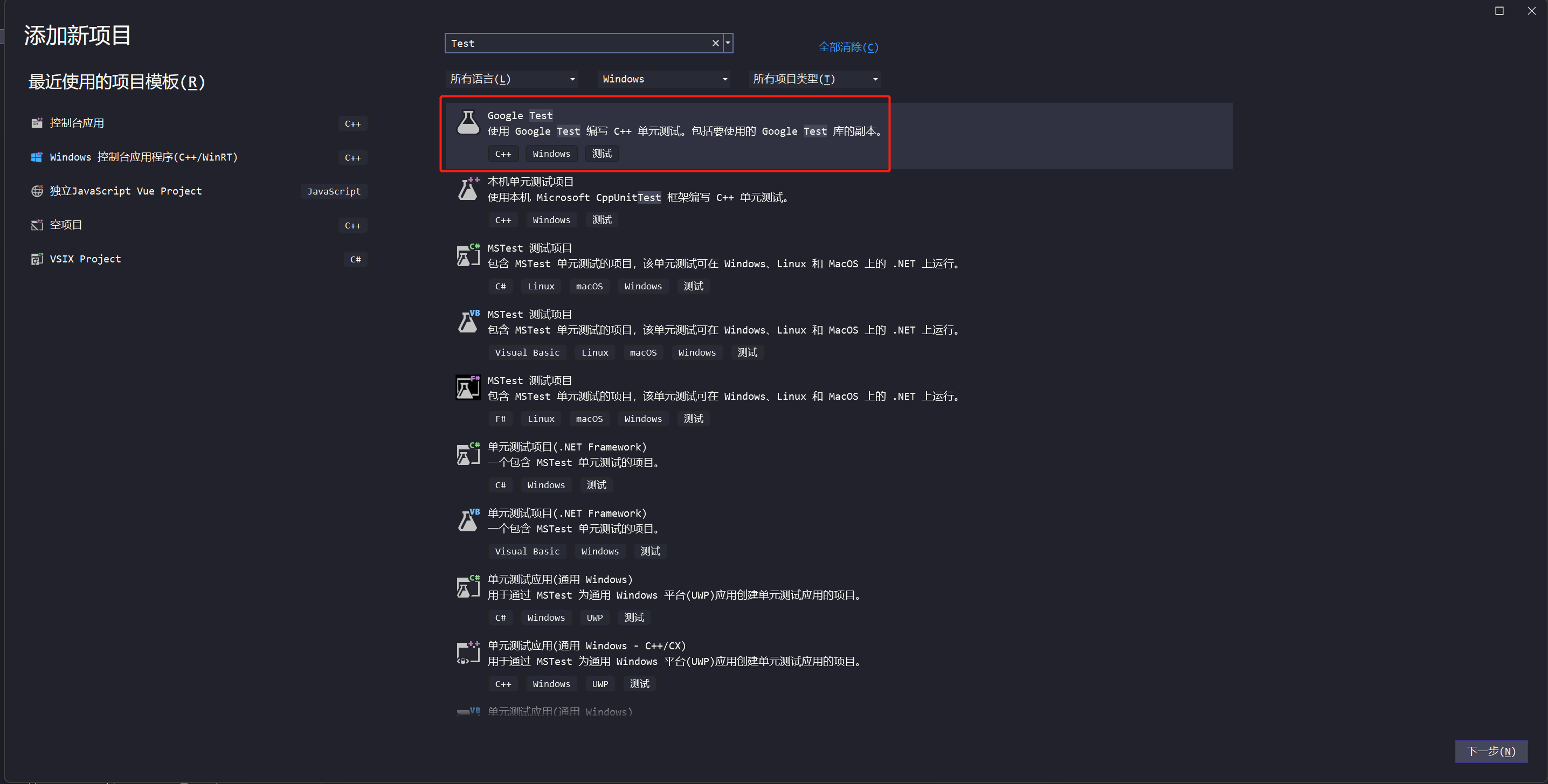
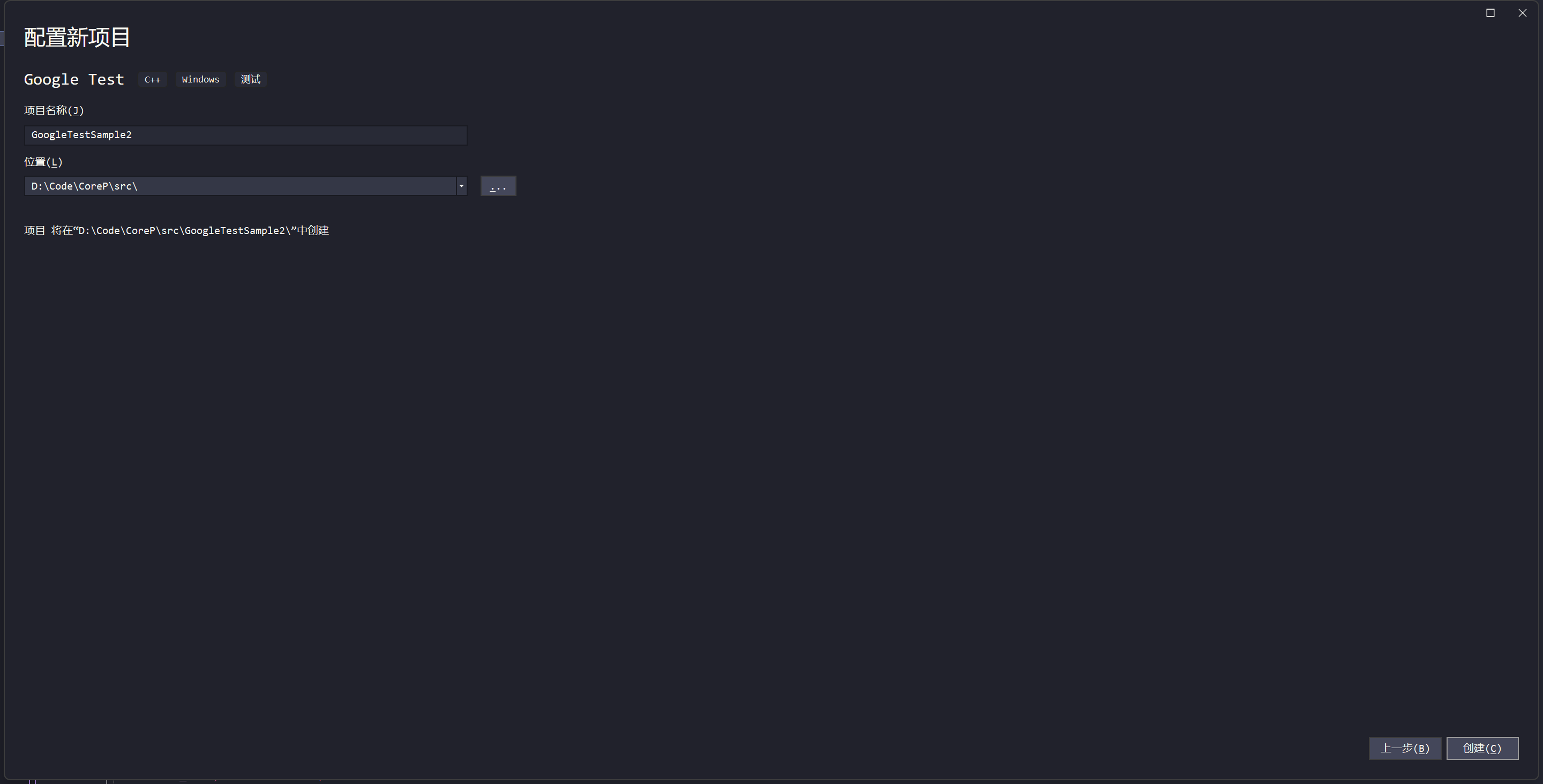
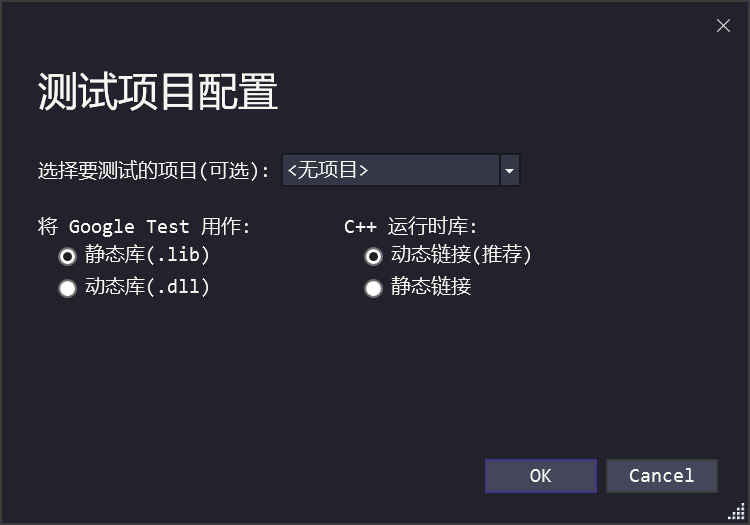
这里目标测试项目填空,可自行添加需要测试的文件。
创建项目后,右键项目属性可发现自动引入了Google Test
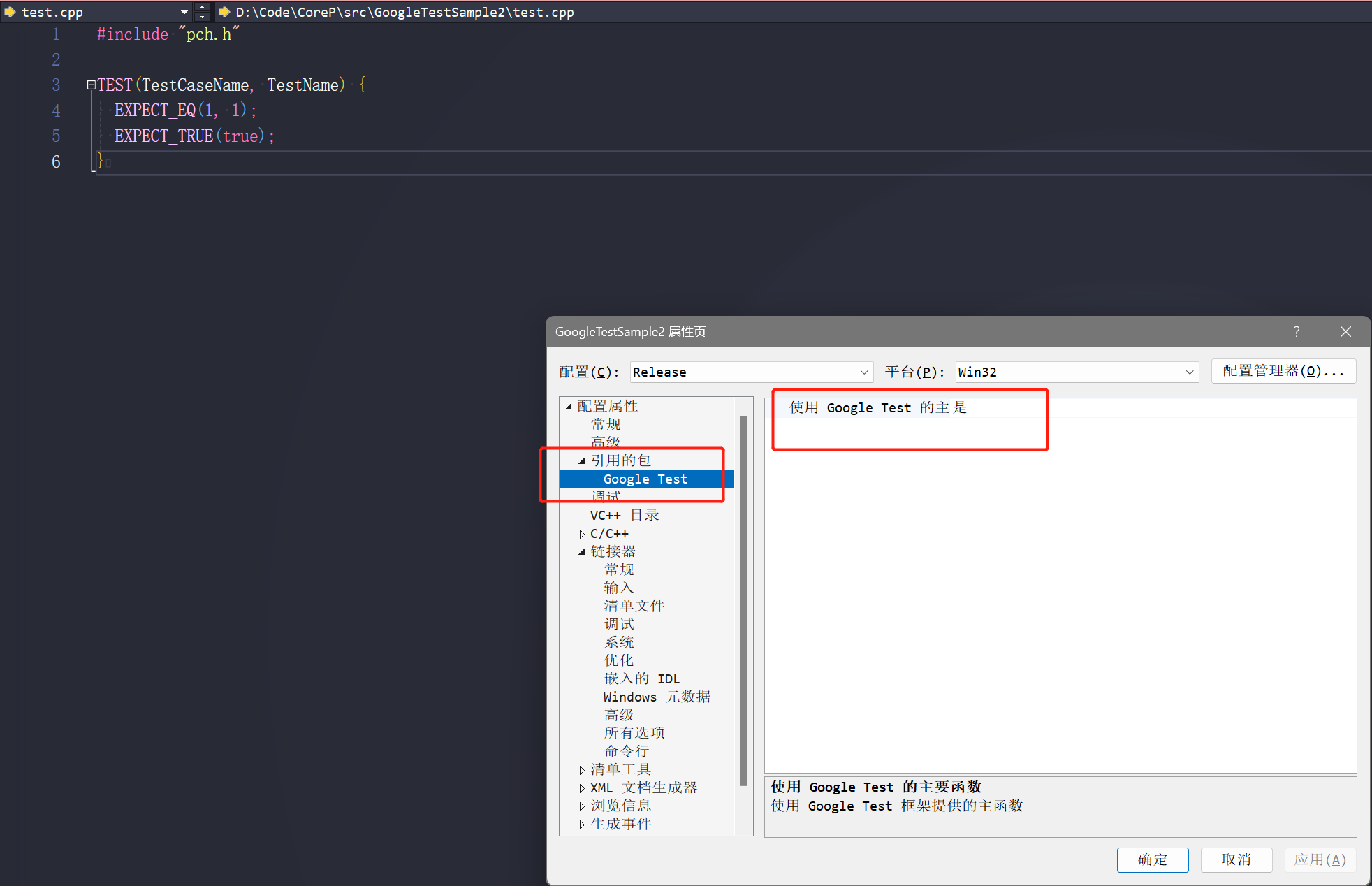
查看项目文件列表,是如下结构
文件名 |
说明 |
内容 |
---|---|---|
pch.cpp/pch.h |
预编译头,引入gtest.h |
#pragma once |